PySide (Qt for Python) は、Qt(キュート)の Python バインディングで、GUI などを構築するためのクロスプラットフォームなライブラリです。Linux/X11, macOS および Microsoft Windows をサポートしています。配布ライセンスは LGPL で公開されています。
Qt ライブラリの機能は GUI 部品にとどまらず、とても広範なので、使ったことの無い機能がたくさんあります。今回は、そのうち、動画再生に利用できる QMediaPlayer で MP4 ファイルを再生する簡単なサンプルを紹介します。
下記の OS 環境で動作確認をしています。
![]() |
Fedora Workstation 39 | x86_64 |
Python | 3.12.1 | |
PySide6 | 6.6.1 |
以下のスクリーンショットは、参考サイト [4] から MP4 ファイルをダウンロードして、それを読み込んで再生している例です。
サンプルは、ツールバーの部分である MyToolBar クラスと、本体 Example クラスに分けました。
サンプルを実行すると下記のようなメッセージが出ます。メッセージが出ても MP4 のファイルの再生はできているのですが、メッセージの解消方法が判れば追記します。
(venv) $ python qt_mediaplayer.py qt.multimedia.ffmpeg.libsymbolsresolver: Couldn't load VAAPI library
このサンプルは、MP4 ファイルを読み込んで、再生するだけのものです。
サンプルの大まかな流れは、まず QMediaPlayer のインスタンス self.player を作成し、QAudioOutput で音、QVideoWidget で動画の出力先のインスタンスをそれぞれ作成して self.player に登録します。
self.player = QMediaPlayer() audio = QAudioOutput(self) self.player.setAudioOutput(audio) video = QVideoWidget(self) self.player.setVideoOutput(video)
MP4 ファイル filename を self.player に読み込んで play メソッドで再生します。
self.player.setSource(QUrl.fromLocalFile(filename)) self.player.play()
QMediaPlayer の機能を試しながら、もっと使いこなせるようにしていきたいです。
参考サイト
- QMediaPlayer - Qt for Python
- QAudioOutput - Qt for Python
- QVideoWidget - Qt for Python
- Download Sample Videos / Dummy Videos For Demo Use
- Video Overview | Qt Multimedia 6.6.1

にほんブログ村
#オープンソース
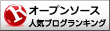

0 件のコメント:
コメントを投稿