PySide (Qt for Python) は、Qt(キュート)の Python バインディングで、GUI などを構築するためのクロスプラットフォームなライブラリです。配布ライセンスは LGPL で公開されています(商用ライセンスも有り)。最新のバージョンは Qt6 に対応した PySide6(記事執筆時点で 6.6.0)です。
ウィジェットのレイアウトの左上に寄せること、これは簡単なことなのにいつまで経っても覚えらず、期待していた配置にならずにあたふたとしてしまうことのひとつです。そういうおバカな自分のための備忘録です。
他のウィジェットなどの都合でウィンドウのサイズが決まっている時に、なにも考えずに例えば QGridLayout でウィジェットを配置します。すると以下のようになってしまって、どうしたら左上にウィジェットを寄せられるのか考え込んでしまうときがあります。
qt_gridlayout_none.py の実行例
qt_gridlayout_none.py
結局、インターネットで調べて、レイアウトのインスタンスを左上に寄せればよいことを見つけて、ああそうだったと思うことになるのです。
layout.setAlignment(Qt.AlignmentFlag.AlignTop | Qt.AlignmentFlag.AlignLeft)
この一行をレイアウトのインスタンスを定義した後に加えるだけで、全体が左上に寄ります。
qt_gridlayout_top_left.py の実行例
qt_gridlayout_top_left.py
念のため、QVBoxLayout でも確認しました。まずは何も考慮しなかった場合です。
qt_vboxlayout_none.py の実行例
qt_vboxlayout_none.py
QGridLayout の時と同様にレイアウトのインスタンスを定義した後に一行加えます。
qt_vboxlayout_top_left.py の実行例
qt_vboxlayout_top_left.py
知っている人から見れば取るに足らないことだとは思います。しかし、自分は以前、Tcl/Tk でながらく GUI アプリを作ってきたので、その時の挙動との違いが覚えにくさを招いているのかもしれないなと考えています。
参考サイト
- python - How do I add widgets to the top left of Pyside Qt layout instead of having the items centered and evenly spaced? - Stack Overflow [2023-03-13]

にほんブログ村
#オープンソース
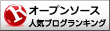

0 件のコメント:
コメントを投稿