PySide (Qt for Python) は、Qt(キュート)の Python バインディングで、GUI などを構築するためのクロスプラットフォームなライブラリです。配布ライセンスは LGPL で公開されています。最新のバージョンは Qt6 に対応した PySide6(記事執筆時点で 6.4.2)です。
参考サイト [1] に QThreadPool と QRunnable を利用したマルチスレッディングの記事が判り易くまとめられていたので、早速記事を参考にしながら自分でもサンプルを作ってみました。
下記の OS 環境で動作確認をしました。
![]() |
Fedora Linux 37 | (Server Edition) | x86_64 |
python3.11 | python3-3.11.1-3.fc37.x86_64 | ||
PySide6 | 6.4.2 |
qt_threadpool.py
別スレッド側では簡単な処理しかしていませんが、QThread でゴリゴリと記述するのに比べると、QThreadPool と QRunnable を利用すると、随分とスッキリ記述できました。
ただ、QRunnable と Signal を多重継承ができなかったので、Signal 用に別クラスを作らなければならなかったのが、ちょっと面倒なことぐらいです。
このサンプルでは START ボタンを続けてクリックすれば、スレッドが次々できますが、そのようなマルチスレッディングが必要な場面は、自分の用途ではとりあえずありません。むしろ、スレッドが処理している間は、同じ処理のスレッドができないように管理しなければならない場面のほうが多そうです。
参考サイト
- Multithreading PySide6 applications with QThreadPool [2022-08-11]
- QThreadPool - Qt for Python
- QRunnable - Qt for Python

にほんブログ村
#オープンソース
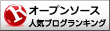

0 件のコメント:
コメントを投稿